Apply for Financing
The Apply mutation is where you actually apply the applicant for financing.
Integration Types
Depending on your integration type there are two different types of applications you can submit, an e-commerce (ecomm) application and a brick and mortar (B&M) application. The integration type is configured on a partner level, so don't need to worry about choosing between the two as applications come in. If you are unsure which type of application you are submitting you can reach out to our team at [email protected].
E-commerce (Ecomm) Application: an ecomm application is an application that will be completed from start to finish online via our APIs. This application cannot be resumed from any other Koalafi merchant systems and must be conducted 100% through our APIs or our modal. This is the most common type of app and should be used unless you have talked to the Koalafi team about doing a B&M integration.
Brick & Mortar (B&M) Application: a B&M application is an application that is created through our APIs and then can be finished either through our APIs or completing the application in the Koalafi dealer portal.
The main difference between these two applications is the inputs you provide when applying the applicant. The different sections below walk through an overview of using the apply mutation, creating the inputs for an ecomm or B&M app, getting an approval and handling errors.
Apply Overview
Schema Documentation
Note this is an example mutation, to see full schema use the schema docs located within the GraphQL playground here.
An example of a condensed apply mutation might look something like:
mutation apply($applyInput: ApplyInput!) {
apply(input: $applyInput) {
application {
lease {
id
status
amount
options {
leaseTerm
approvedAmount
}
}
customer {
id
emailAddress
cellPhone
firstName
lastName
}
}
applicationErrors {
__typename
... on DenialError {
message
}
... on FatalError {
message
}
... on BadRequest {
message
}
... on HardDecline {
message
}
... on SoftDecline {
message
extensions {
declineField
appId
}
}
... on LeaseRestricted {
message
}
... on NotFound {
message
}
... on Ineligible {
message
}
... on CartValidation {
message
extensions {
validationErrType
approvalType
approvedAmount
}
}
}
}
}
When writing your mutation, you will want to return more fields than included in this example so that you get as much information about an approved application as possible. Note, some fields may return as null on apply.
Choosing the Input
The input you pass to the mutation will also depend on whether or not you are apply with an e-commerce application or a brick and mortar application.
E-commerce (Ecomm) Application: an ecomm application is an application that will be completed from start to finish online via our APIs. This application cannot be resumed from any other Koalafi merchant systems and must be conducted 100% through our APIs. It has a smaller required apply input than the brick and mortar application. This is the most common type of app and should be used unless you have talked to the Koalafi team about doing a B&M integration.
Brick & Mortar (B&M) Application: a B&M application is an application that is created through our APIs and then can be finished either through our APIs or completing the application in the Koalafi dealer portal. This type of application requires more information to be sent in the apply input in order to resume the app in our downstream systems.
The below sections walk through the difference in apply inputs as well as the changes to the application flow based on your integration type.
Including Items
Regardless of the type of application you are submitting, items are optional to include in the apply input. Many times you may be approving a customer before they have started shopping or without access to their shopping part. You only need to include items if you have access to them at the time they apply.
However, if a customer changes their cart prior to checkout or if you did not include the items on apply then you will need to update the Koalafi order with items prior to checkout. There are instructions on how to update the Koalafi order to match the customer's cart in our Lease Approval & Checkout sections later in the docs and include instructions for both an e-comm app and a B&M app.
Apply for E-commerce Application
Input
An example input for an e-commerce application is as follows:
{
"applyInput": {
"customer": {
"firstName": "Wally",
"lastName": "Koala",
"cellPhone": "5555555555",
"emailAddress": "[email protected]",
"taxpayerIdentificationNumber": "110332232",
"birthDate": "1999-01-01",
"billingAddress": {
"line1": "424 Hull St",
"line2": null,
"city": "Richmond",
"state": "VA",
"zip": "23224"
},
},
"employment": {
"monthlyIncome": "6000",
},
"orderId": "041855ff-2910-4506-a56c-53763d660dfb"
}
}
All of the above fields will be marked as required in our API schema docs in our playground. Our API validates that all the required fields are passed into the mutation and if one is missing you will see the following validation error returned:
{
"errors": [
{
"message": "employment must be defined",
"path": [
"apply",
"employment"
]
},
{
"message": "validation Error",
"path": [
"apply"
]
}
],
"data": null
}
If you get a validation error, you can fix the input and resubmit the apply mutation.
Flows
The apply mutation can result in a many different outcomes
- Lease Approval
- Soft Decline
- Hard Decline
- Approval with Cart Validation Errors
- Other Application Errors
The below flow diagram visualizes the possible application flow for an ecomm app and our sections below walk through how to recognize the different scenarios and the next steps.
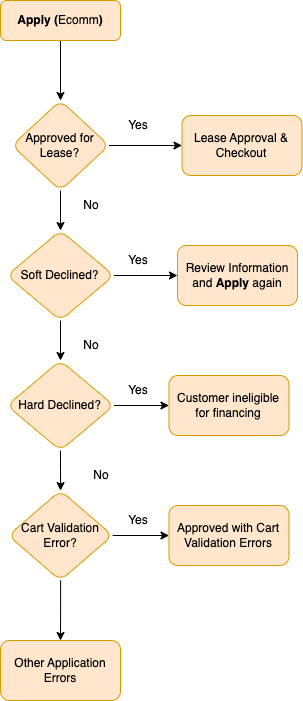
Apply for Brick and Mortar Application
A brick and mortar application requires more fields to be passed into apply than an e-commerce app.
Prequalification Applications
Not all fields are required. Optional fields are notated in the JSON below
Inputs
An example input for a brick and mortar application looks like
{
"applyInput": {
"customer": {
"firstName": "Wally",
"lastName": "Koala",
"cellPhone": "5555555555",
"emailAddress": "[email protected]",
"taxpayerIdentificationNumber": "110332232",
"birthDate": "1999-01-01",
"billingAddress": {
"line1": "424 Hull St",
"line2": null,
"city": "Richmond",
"state": "VA",
"zip": "23224"
},
},
"employment": {
"monthlyIncome": "6000",
"payPeriod": "monthly", // Not required for prequal
"lastPayDate": "2022-11-11", // Not required for prequal
"isPaidViaDirectDeposit": true // Not required for prequal
},
"bankAccount": { // Not required for prequal (entire object)
"routingNumber": "011000028",
"accountNumber": "12345"
},
"paymentInfo": { // Not required for prequal (entire object)
"cardholderFirstName": "Wally",
"cardholderLastName": "Koala",
"cardNumber": "5475776082417759",
"expirationDate": "2025-02",
"billingZip": "11111",
"billingState": "VA"
},
"orderId": "041855ff-2910-4506-a56c-53763d660dfb" // optional, orderId will be created for you and returned if not passed in
}
}
Our API validates that the input has all the required fields for a brick and mortar app and will return a detailed message with any missing fields. This is an example of the validation error response:
{
"errors": [
{
"message": "bankAccount must be defined",
"path": [
"apply",
"bankAccount"
]
},
{
"message": "paymentInfo must be defined",
"path": [
"apply",
"paymentInfo"
]
},
{
"message": "payPeriod must be defined",
"path": [
"apply",
"employment",
"payPeriod"
]
},
{
"message": "lastPayDate must be defined",
"path": [
"apply",
"employment",
"lastPayDate"
]
},
{
"message": "isPaidViaDirectDeposit must be defined",
"path": [
"apply",
"employment",
"isPaidViaDirectDeposit"
]
},
{
"message": "validation Error",
"path": [
"apply"
]
}
],
"data": null
}
Flows
The apply mutation can result in a many different outcomes:
- Lease Approval
- Soft Decline
- Hard Decline
- Approval with Cart Validation Errors
- Other Application Errors
The below flow diagram visualizes the possible application flow for a B&M app and our sections below walk through how to recognize the different scenarios and the next steps.
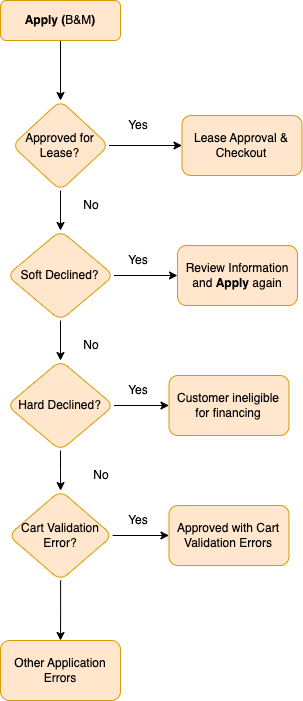
Check for Errors
You should always check for errors first before checking for an application success. There are many different errors that can be returned from this mutation, but the most important ones to look out for are hardDecline, softDecline and cartValidation. The sections below explain what these errors mean for the customer and the next steps for the app.
For any other application errors returned you can find more information on Handling Common Errors.
Hard Decline
In this scenario an applicant has been denied for Koalafi financing at this time and cannot resubmit their application. The applicant can expect to receive more information about the decline in the mail.
Soft Decline
A soft decline is returned when there is an error with the application and we are not able to approve their application at this time. This is normally due to an issue with the information the customer entered, but the decline field will give more information about why the applicant was declined. If a customer updates the information on their application, then the application can be resubmitted.
{
"__typename": "SoftDecline",
"message": "Customer was soft declined",
"extensions": {
"declineField": "application was soft declined due to X reason",
"appId": 123456710,
}
}
Even though our decline field says why a customer was soft declined, to help protect against fraud we recommend keeping the error message shown to the customer vague. For example, instead of saying "Please check your SSN and resubmit your application" we suggest something like "Please verify your personal information and resubmit your application." This ensures that we don't accidentally give a bad actor the information they need to submit a fraudulent application.
Resolution
The resolution for a soft decline is the same for both ecomm and B&M applications:
- Let the customer know there was an issue with their application and encourage them to verify the information they provided on their application.
- Once their application details have been updated, use the apply mutation to resubmit their application.
- Check the response like you would for the initial apply request.
It's possible that resubmitting the app could result in another soft-decline. In that case, repeat the steps outline in this section. However, after 3 retries on a soft-declined application, the app is automatically hard declined.
Approved with Cart Validation Errors
A cartValidation error is only returned when you send items in the apply input. This error means that a customer is approved for financing but their cart does not meet the requirements to continue.
A cartValidation error will look something like:
{
"__typename": "CartValidation",
"message": "Customer was approved, but their cart total exceeds approval amount",
"extensions": {
"validationErrType": "CartTotalExceedsApproval",
"approvalType": "lease",
"approvedAmount": "3750"
}
}
You will want to make sure that your mutation has included all three fields in the cartValidation error extension as validationErrType
approvalType
and approvedAmount
hold important information to help you resolve the error.
The fields you want to include in your mutation are as follows:
... on CartValidation {
message
extensions {
validationErrType
approvalType
approvedAmount
}
}
validationErrType
indicates the type of cart validation encountered with the appapprovalType
lets you know whether the customer was approved for a leaseapprovedAmount
indicates how much the customer was approved for
More information about this error can be found on our Handling Common Errors page.
Ecomm Resolution
If you are submitting an ecomm application and not using our modal, then you we recommend the following steps for resolution:
- Show the customer that they have been approved for a lease and the approval amount but that there is an issue with their cart
- Using the
validationErrType
let the customer know how to modify their cart so they can continue to checkout with Koalafi. For example, if they have exceeded their approval amount you might show a message like "Congratulations, you've been approved for a lease. Your current cart total of $4,500 exceeds your approval amount of $4000. Please remove items from your cart to continue to checkout”. - Once they have modified their cart, call UpdateOrderItems mutation to sync the Koalafi order with the customer cart. More details on this mutation are found on the Lease Approval & Checkout (Ecomm) pages.
If you are using our modal post-apply then you don't need to take any action at this time as our UI will display an error message.
B&M Resolution
If you are submitting a B&M application and switching to our merchant portal after Apply, then you we recommend the following steps for resolution:
- Show the customer that they have been approved for a lease and the approval amount but that there is an issue with their cart
- Using the
validationErrType
let the customer know how to modify their cart so they can continue to checkout with Koalafi. For example, if they have exceeded their approval amount you might show a message like "Congratulations, you've been approved for a lease. Your current cart total of $4,500 exceeds your approval amount of $4000”.- The customer can work with the merchant to adjust their cart within the Koalafi merchant portal
If you are submitting a B&M application but continuing to use our APIs for the duration of the application process, then you will need to do one more step.
- Once the customer has modified their cart, call UpdateOrderItems mutation to sync the Koalafi order with the customer cart. More details on this mutation are found on the Lease Approval & Checkout (Ecomm) pages.
Other Application Errors
For more information on our other, less frequently returned application Errors see our Handling Common Errors page.
Check for Approved Application
An approved application response will look something like this:
"data":{
"apply"{
"application" : {
"lease": Lease | null,
"customer": Customer
},
"applicationErrors": [] | null
}
}
The most important thing in this response is that applicationErrors
is either empty or null and application
is not. This indicates the mutation was successful and that you have an approved application. In order to determine whether the customer was approved for a lease, you will need to look at the application
object.
Lease Approval
To identify a lease approval you'll need to look at the returned application
object. An example lease approval response will look something like:
"data": {
"apply": {
"application": {
"lease": {
"id": 30785,
"status": "preApproved",
"draftStep": "reviewAndSubmit",
"maxLeaseAmount": "4600",
"options": [
{
"leaseTerm": "24",
"approvedAmount": "4600",
"applicationFee": "40",
"earlyBuyoutDiscount": "50",
"sacTerm": 90
},
{
"leaseTerm": "12",
"approvedAmount": "3000",
"applicationFee": "40",
"earlyBuyoutDiscount": "35",
"sacTerm": 90
}
],
"displayId": "71896-2"
},
"customer": {
"emailAddress": "[email protected]",
"cellPhone": "5555555555",
"firstName": "Wally",
"lastName": "Koala"
}
},
"applicationErrors": []
}
}
You will need to make note of the lease id
returned from this mutation as it is needed to finish the financing process.
If you have a lease approval and applied as an ecomm app, you can continue to Lease Approval & Checkout (Ecomm) page. If you have a lease approval and applied as a B&M app you can continue to the Lease Approval & Checkout (B&M) page.
Updated 11 days ago
Continue on to our Approval & Checkout pages to see the remaining steps within the Koalafi financing process.